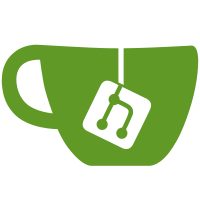
* Added Dynamic Gravity Toggle Added an editor toggle for dynamic gravity so users don't need to uncomment code in character.gd. * Refactor controls export variables Changed controls export variables to be a single dictionary so they can be edited completely in the editor without having to open the script. I also refactored the controller support section to allow similar functionality for controller specific controls and removed the need to uncomment/comment out lines of code to turn on/off controller support. * Add more editor descriptions and implement camera axis inversion options Added/tweaked export variable descriptions so everything has one. Also slightly refactored the camera input inversion code and added the ability to invert the camera x axis. * Added direction based landing animations Added some logic to determine which landing animation to play so it's not random anymore. Also added a land_center animation for the case of neutral landings. This can be expanded if desired, but it's pretty convincing as is and is ulitmately a small detail. * Correct various grammar and spelling errors A final pass to clean up any grammatical or spelling errors in the comments * Re-organized Character.gd Hope I'm not overstepping here, but I re-organized Character.gd to make it a bit easier to read and work with. Added editor bookmarks and collapsible code regions. It's hard to parse in the diff so I recommend you look at it in Godot proper. * Commented out debug print statement I assume this was left uncommented by mistake. I was wondering what was printing that to my console. * Fixed Landing Animation Logic I guess I was up too late on my first attempt because it straight up didn't work. This new version works as far as my testing can tell. * Removed some unused variables
50 lines
1.4 KiB
GDScript
50 lines
1.4 KiB
GDScript
@tool
|
|
extends Node
|
|
|
|
# This does not effect runtime yet but will in the future.
|
|
|
|
@export_category("Controller Editor Module")
|
|
@export_range(-360.0, 360.0, 0.01, "or_greater", "or_less") var head_y_rotation : float = 0.0:
|
|
set(new_rotation):
|
|
if HEAD:
|
|
head_y_rotation = new_rotation
|
|
HEAD.rotation.y = deg_to_rad(head_y_rotation)
|
|
update_configuration_warnings()
|
|
@export_range(-90.0, 90.0, 0.01, "or_greater", "or_less") var head_x_rotation : float = 0.0:
|
|
set(new_rotation):
|
|
if HEAD:
|
|
head_x_rotation = new_rotation
|
|
HEAD.rotation.x = deg_to_rad(head_x_rotation)
|
|
update_configuration_warnings()
|
|
|
|
@export_group("Nodes")
|
|
@export var CHARACTER : CharacterBody3D
|
|
@export var head_path : String = "Head" # Relative to the parent node
|
|
#@export var CAMERA : Camera3D
|
|
#@export var HEADBOB_ANIMATION : AnimationPlayer
|
|
#@export var JUMP_ANIMATION : AnimationPlayer
|
|
#@export var CROUCH_ANIMATION : AnimationPlayer
|
|
#@export var COLLISION_MESH : CollisionShape3D
|
|
|
|
@onready var HEAD = get_node("../" + head_path)
|
|
|
|
|
|
func _ready():
|
|
if !Engine.is_editor_hint():
|
|
#print("not editor")
|
|
HEAD.rotation.y = deg_to_rad(head_y_rotation)
|
|
HEAD.rotation.x = deg_to_rad(head_x_rotation)
|
|
|
|
|
|
func _get_configuration_warnings():
|
|
var warnings = []
|
|
|
|
if head_y_rotation > 360:
|
|
warnings.append("The head rotation is greater than 360")
|
|
|
|
if head_y_rotation < -360:
|
|
warnings.append("The head rotation is less than -360")
|
|
|
|
# Returning an empty array gives no warnings
|
|
return warnings
|