mirror of
git://git.tartarus.org/simon/puzzles.git
synced 2025-04-21 08:01:30 -07:00
Files
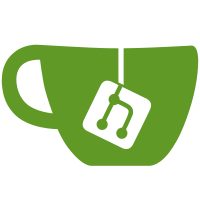
I had this idea today and immediately wondered why I'd never had it before! To generate the puzzle screenshots used on the website and as program icons, we run the GTK front end with the --screenshot option, which sets up GTK, insists on connecting to an X server (or other display), draws the state of a puzzle on a Cairo surface, writes that surface out to a .png file, and exits. But there's no reason we actually need the GTK setup during that process, especially because the surface we do the drawing on is our _own_ surface, not even one provided to us by GTK. We could just set up a Cairo surface by itself, draw on it, and save it to a file. Calling gtk_init is not only pointless, but actively inconvenient, because it means the build script depends on having an X server available for the sole purpose of making gtk_init not complain. So now I've simplified things, by adding a 'headless' flag in new_window and the frontend structure, which suppresses all uses of actual GTK, leaving only the Cairo surface setup and enough supporting stuff (like colours) to generate the puzzle image. One awkward build dependency removed. This means that --screenshot no longer works in GTK 2, which I don't care about, because it only needs to run on _one_ platform.
237 lines
11 KiB
Bash
237 lines
11 KiB
Bash
# -*- sh -*-
|
|
# Build script to build Puzzles.
|
|
#
|
|
# You can cut out large components of the build by defining a subset
|
|
# of these options on the bob command line:
|
|
# -DNOSIGN -DNOWINDOWS -DNOMACOS -DNOICONS -DNOJAVA -DNOJS
|
|
|
|
module puzzles
|
|
|
|
set Version $(!builddate).$(vcsid)
|
|
|
|
# Start by substituting the right version number in configure.ac.
|
|
in puzzles do perl -i~ -pe 's/6.66/$(Version)/' configure.ac
|
|
in puzzles do rm configure.ac~
|
|
|
|
# And put it into the documentation as a versionid.
|
|
# use perl to avoid inconsistent behaviour of echo '\v'
|
|
in puzzles do perl -e 'print "\n\\versionid Simon Tatham'\''s Portable Puzzle Collection, version $$ARGV[0]\n"' $(Version) >> puzzles.but
|
|
in puzzles do perl -e 'print "\n\\versionid Simon Tatham'\''s Portable Puzzle Collection, version $$ARGV[0]\n"' $(Version) >> devel.but
|
|
|
|
# Write out a version.h that contains the real version number.
|
|
in puzzles do echo '/* Generated by automated build script */' > version.h
|
|
in puzzles do echo '$#define VER "Version $(Version)"' >> version.h
|
|
|
|
# And do the same substitution in the OS X metadata. (This is a bit
|
|
# icky in principle because it presumes that my version numbers don't
|
|
# need XML escaping, but frankly, if they ever do then I should fix
|
|
# them!)
|
|
in puzzles do perl -i -pe 's/Unidentified build/$(Version)/' osx-info.plist
|
|
|
|
ifneq "$(NOICONS)" yes then
|
|
# First build some local binaries, to run the icon build.
|
|
in puzzles do perl mkfiles.pl -U CFLAGS='-Wwrite-strings -Werror'
|
|
in puzzles do make -j$(nproc)
|
|
|
|
# Now build the screenshots and icons.
|
|
in puzzles/icons do make web winicons gtkicons -j$(nproc)
|
|
|
|
# Destroy the local binaries and autoconf detritus, mostly to avoid
|
|
# wasting network bandwidth by transferring them to the delegate
|
|
# servers.
|
|
in puzzles do make distclean
|
|
|
|
endif
|
|
|
|
# Re-run mkfiles.pl now that it knows the icons are there. (Or for the
|
|
# first time, if we didn't bother building the icons.)
|
|
in puzzles do perl mkfiles.pl
|
|
|
|
# Rebuild the configure script.
|
|
in puzzles do ./mkauto.sh
|
|
|
|
ifneq "$(NOMACOS)" yes then
|
|
# Build the OS X .dmg archive.
|
|
delegate osx
|
|
in puzzles do make -f Makefile.osx clean
|
|
in puzzles do make -f Makefile.osx release VER=-DVER=$(Version) XFLAGS='-Wwrite-strings -Werror' -j$(nproc)
|
|
return puzzles/Puzzles.dmg
|
|
enddelegate
|
|
endif
|
|
|
|
ifneq "$(NOWINDOWS)" yes then
|
|
# Build the Windows binaries and installer, and the CHM file.
|
|
in puzzles do make -f Makefile.doc clean
|
|
in puzzles do make -f Makefile.doc -j$(nproc) # build help files for installer
|
|
in puzzles do mason.pl --args '{"version":"$(Version)","descfile":"gamedesc.txt"}' winwix.mc > puzzles.wxs
|
|
in puzzles do perl winiss.pl $(Version) gamedesc.txt > puzzles.iss
|
|
ifneq "$(VISUAL_STUDIO)" "yes" then
|
|
in puzzles with clangcl64 do mkdir win64 && Platform=x64 make -f Makefile.clangcl BUILDDIR=win64/ VER=-DVER=$(Version) XFLAGS='-Wwrite-strings -Werror' -j$(nproc)
|
|
in puzzles with clangcl32 do mkdir win32 && Platform=x86 make -f Makefile.clangcl BUILDDIR=win32/ SUBSYSVER=,5.01 VER=-DVER=$(Version) XFLAGS='-Wwrite-strings -Werror' -j$(nproc)
|
|
# Code-sign the binaries, if the local bob config provides a script
|
|
# to do so. We assume here that the script accepts an -i option to
|
|
# provide a 'more info' URL, and an optional -n option to provide a
|
|
# program name, and that it can take multiple .exe filename
|
|
# arguments and sign them all in place.
|
|
ifneq "$(cross_winsigncode)" "" in puzzles do $(cross_winsigncode) -i https://www.chiark.greenend.org.uk/~sgtatham/puzzles/ win64/*.exe win32/*.exe
|
|
# Build installers.
|
|
in puzzles with wixonlinux do candle -arch x64 puzzles.wxs -dWin64=yes -dBindir=win64/ && light -ext WixUIExtension -sval puzzles.wixobj
|
|
in puzzles with wixonlinux do candle -arch x86 puzzles.wxs -dWin64=no -dBindir=win32/ && light -ext WixUIExtension -sval puzzles.wixobj -o puzzles32.msi
|
|
ifneq "$(cross_winsigncode)" "" in puzzles do $(cross_winsigncode) -i https://www.chiark.greenend.org.uk/~sgtatham/puzzles/ -n "Simon Tatham's Portable Puzzle Collection Installer" puzzles.msi puzzles32.msi
|
|
else
|
|
delegate windows
|
|
in puzzles with visualstudio do/win nmake -f Makefile.vc clean
|
|
in puzzles with visualstudio do/win nmake -f Makefile.vc VER=-DVER=$(Version)
|
|
ifneq "$(winsigncode)" "" in puzzles do $(winsigncode) -i https://www.chiark.greenend.org.uk/~sgtatham/puzzles/ win64/*.exe
|
|
# Build installers.
|
|
in puzzles with wix do/win candle puzzles.wxs -dWin64=yes -dBindir=win64/ && light -ext WixUIExtension -sval puzzles.wixobj
|
|
in puzzles with innosetup do/win iscc puzzles.iss
|
|
return puzzles/win64/*.exe
|
|
return puzzles/puzzles.msi
|
|
enddelegate
|
|
endif
|
|
in puzzles do chmod +x win32/*.exe win64/*.exe
|
|
endif
|
|
|
|
# Build the Pocket PC binaries and CAB.
|
|
#
|
|
# NOTE: This part of the build script requires the Windows delegate
|
|
# server to have the cabwiz program on its PATH. This will
|
|
# typically be at
|
|
#
|
|
# C:\Program Files\Windows CE Tools\WCE420\POCKET PC 2003\Tools
|
|
#
|
|
# but it might not be if you've installed it somewhere else, or
|
|
# have a different version.
|
|
#
|
|
# NOTE ALSO: This part of the build is commented out, for the
|
|
# moment, because cabwiz does unhelpful things when run from within
|
|
# a bob delegate process (or, more generally, when run from any
|
|
# terminal-based remote login to a Windows machine, including
|
|
# Cygwin opensshd and Windows Telnet). The symptom is that cabwiz
|
|
# just beeps and sits there. Until I figure out how to build the
|
|
# .cab from an automated process (and I'm willing to consider silly
|
|
# approaches such as a third-party CAB generator), I don't think I
|
|
# can sensibly enable this build.
|
|
|
|
#in puzzles do perl wceinf.pl gamedesc.txt > puzzles.inf
|
|
#delegate windows
|
|
# in puzzles do cmd /c 'wcearmv4 & nmake -f Makefile.wce clean'
|
|
# in puzzles do cmd /c 'wcearmv4 & nmake -f Makefile.wce VER=-DVER=$(Version)'
|
|
# # Nasty piece of sh here which saves the return code from cabwiz,
|
|
# # outputs its errors and/or warnings, and then propagates the
|
|
# # return code back to bob. If only cabwiz could output to
|
|
# # standard error LIKE EVERY OTHER COMMAND-LINE UTILITY IN THE
|
|
# # WORLD, I wouldn't have to do this.
|
|
# in puzzles do cat puzzles.inf
|
|
# in puzzles do cmd /c 'wcearmv4 & bash -c cabwiz puzzles.inf /err cabwiz.err /cpu ARMV4'; a=$$?; cat cabwiz.err; exit $$a
|
|
# return puzzles/puzzles.armv4.cab
|
|
#enddelegate
|
|
|
|
# Build the HTML docs.
|
|
in puzzles do mkdir doc
|
|
in puzzles do mkdir devel
|
|
in puzzles/doc do halibut --html -Chtml-contents-filename:index.html -Chtml-index-filename:indexpage.html -Chtml-template-filename:%k.html -Chtml-template-fragment:%k ../puzzles.but
|
|
in puzzles/devel do halibut --html -Chtml-contents-filename:index.html -Chtml-index-filename:indexpage.html -Chtml-template-filename:%k.html -Chtml-template-fragment:%k ../devel.but
|
|
|
|
ifneq "$(NOWINDOWS)" yes then
|
|
# Move the deliver-worthy Windows binaries (those specified in
|
|
# gamedesc.txt, which is generated by mkfiles.pl and helpfully
|
|
# excludes the command-line auxiliary utilities such as solosolver,
|
|
# and nullgame.exe) into a subdirectory for easy access.
|
|
in puzzles do mkdir winbin64 winbin32
|
|
in puzzles/win64 do mv `cut -f2 -d: ../gamedesc.txt` ../winbin64
|
|
in puzzles/win32 do mv `cut -f2 -d: ../gamedesc.txt` ../winbin32
|
|
|
|
# Make a zip file of the Windows binaries and help files.
|
|
in puzzles do zip -j puzzles.zip winbin64/*.exe puzzles.chm puzzles.hlp puzzles.cnt
|
|
in puzzles do zip -j puzzles32.zip winbin32/*.exe puzzles.chm puzzles.hlp puzzles.cnt
|
|
endif
|
|
|
|
# Create the source archive. (That writes the archive into the
|
|
# _parent_ directory, so be careful when we deliver it.)
|
|
in puzzles do ./makedist.sh $(Version)
|
|
|
|
# Build the autogenerated pieces of the main web page.
|
|
in puzzles do perl webpage.pl
|
|
|
|
ifneq "$(JAVA_UNFINISHED)" "" in puzzles do perl -i~ -pe 'print "!srcdir unfinished/\n" if /!srcdir icons/' Recipe
|
|
ifneq "$(JAVA_UNFINISHED)" "" in puzzles do ln -s unfinished/group.R .
|
|
ifneq "$(JAVA_UNFINISHED)" "" in puzzles do perl mkfiles.pl
|
|
|
|
ifneq "$(NOJAVA)" yes then
|
|
# Build the Java applets.
|
|
delegate nestedvm
|
|
in puzzles do make -f Makefile.nestedvm NESTEDVM="$$NESTEDVM" VER=-DVER=$(Version) XFLAGS="-Wwrite-strings -Werror" -j$(nproc)
|
|
return puzzles/*.jar
|
|
enddelegate
|
|
endif
|
|
|
|
# Build the Javascript applets. Since my master build machine doesn't
|
|
# have the right dependencies installed for Emscripten, I do this by a
|
|
# delegation.
|
|
ifneq "$(NOJS)" yes then
|
|
in puzzles do mkdir js # so we can tell output .js files from emcc*.js
|
|
delegate emscripten
|
|
in puzzles do make -f Makefile.emcc OUTPREFIX=js/ clean
|
|
in puzzles do make -f Makefile.emcc OUTPREFIX=js/ XFLAGS="-Wwrite-strings -Werror" -j$(nproc)
|
|
return puzzles/js/*.js
|
|
enddelegate
|
|
|
|
# Build a set of wrapping HTML pages for easy testing of the
|
|
# Javascript puzzles. These aren't quite the same as the versions that
|
|
# will go on my live website, because those ones will substitute in a
|
|
# different footer, and not have to link to the .js files with the
|
|
# ../js/ prefix. But these ones should be good enough to just open
|
|
# using a file:// URL in a browser after running a build, and make
|
|
# sure the main functionality works.
|
|
in puzzles do mkdir jstest
|
|
in puzzles/jstest do ../html/jspage.pl --jspath=../js/ /dev/null ../html/*.html
|
|
endif
|
|
|
|
# Set up .htaccess containing a redirect for the archive filename.
|
|
in puzzles do echo "AddType application/octet-stream .chm" > .htaccess
|
|
in puzzles do echo "AddType application/octet-stream .hlp" >> .htaccess
|
|
in puzzles do echo "AddType application/octet-stream .cnt" >> .htaccess
|
|
in . do set -- puzzles*.tar.gz; echo RedirectMatch temp '(.*/)'puzzles.tar.gz '$$1'"$$1" >> puzzles/.htaccess
|
|
in puzzles do echo RedirectMatch temp '(.*/)'puzzles-installer.msi '$$1'puzzles-$(Version)-installer.msi >> .htaccess
|
|
|
|
# Phew, we're done. Deliver everything!
|
|
ifneq "$(NOICONS)" yes then
|
|
deliver puzzles/icons/*-web.png $@
|
|
endif
|
|
ifneq "$(NOWINDOWS)" yes then
|
|
deliver puzzles/winbin64/*.exe $@
|
|
deliver puzzles/winbin32/*.exe w32/$@
|
|
deliver puzzles/puzzles.zip $@
|
|
deliver puzzles/puzzles32.zip w32/$@
|
|
deliver puzzles/puzzles.msi puzzles-$(Version)-installer.msi
|
|
deliver puzzles/puzzles32.msi w32/puzzles-$(Version)-32bit-installer.msi
|
|
deliver puzzles/puzzles.chm $@
|
|
deliver puzzles/puzzles.hlp $@
|
|
deliver puzzles/puzzles.cnt $@
|
|
endif
|
|
deliver puzzles/.htaccess $@
|
|
deliver puzzles/doc/*.html doc/$@
|
|
deliver puzzles/devel/*.html devel/$@
|
|
ifneq "$(NOMACOS)" yes then
|
|
deliver puzzles/Puzzles.dmg $@
|
|
endif
|
|
ifneq "$(NOJAVA)" yes then
|
|
deliver puzzles/*.jar java/$@
|
|
endif
|
|
ifneq "$(NOJS)" yes then
|
|
deliver puzzles/js/*.js js/$@
|
|
deliver puzzles/jstest/*.html jstest/$@
|
|
deliver puzzles/html/*.html html/$@
|
|
deliver puzzles/html/*.pl html/$@
|
|
endif
|
|
deliver puzzles/wwwspans.html $@
|
|
deliver puzzles/wwwlinks.html $@
|
|
|
|
# deliver puzzles/puzzles.armv4.cab $@ # (not built at the moment)
|
|
|
|
# This one isn't in the puzzles subdir, because makedist.sh left it
|
|
# one level up.
|
|
deliver puzzles*.tar.gz $@
|