mirror of
git://git.tartarus.org/simon/puzzles.git
synced 2025-04-20 07:31:30 -07:00
Files
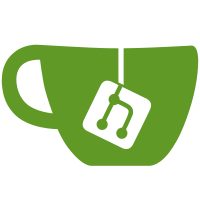
Having stated the principle in the previous commit, I should apply it consistently. A source file linked into the Puzzles library of common support code should not also define a main() under ifdef. This commit only goes as far as the _library_ support modules. It would be a much bigger job to do the same for all the actual _puzzles_ that have test main()s or standalone-solver main()s. And it's not necessary, because modifying one of those source files only triggers a rebuild of _one_ puzzle, not absolutely everything. (Not to mention that it's quite likely the puzzle and the test main() will need to be modified in conjunction anyway.) As in the previous commit, this has required exposing a few internal API functions as global, and maybe editing them a bit. In particular, the one-shot internal function that divvy_rectangle() loops on until it succeeds is now exposed as divvy_rectangle_attempt(), which means the test program doesn't have to condition a failure counter into the real function. I've thrown away penrose-vector-test completely, because that didn't look like a test program with any ongoing use at all - it was surely vestigial, while James was getting the vector representation up and running in the first place.
74 lines
1.3 KiB
C
74 lines
1.3 KiB
C
#include <assert.h>
|
|
#include <string.h>
|
|
|
|
#include "puzzles.h"
|
|
|
|
/* horrific and doesn't check overflow. */
|
|
static long factx(long x, long y)
|
|
{
|
|
long acc = 1, i;
|
|
|
|
for (i = y; i <= x; i++)
|
|
acc *= i;
|
|
return acc;
|
|
}
|
|
|
|
void reset_combi(combi_ctx *combi)
|
|
{
|
|
int i;
|
|
combi->nleft = combi->total;
|
|
for (i = 0; i < combi->r; i++)
|
|
combi->a[i] = i;
|
|
}
|
|
|
|
combi_ctx *new_combi(int r, int n)
|
|
{
|
|
long nfr, nrf;
|
|
combi_ctx *combi;
|
|
|
|
assert(r <= n);
|
|
assert(n >= 1);
|
|
|
|
combi = snew(combi_ctx);
|
|
memset(combi, 0, sizeof(combi_ctx));
|
|
combi->r = r;
|
|
combi->n = n;
|
|
|
|
combi->a = snewn(r, int);
|
|
memset(combi->a, 0, r * sizeof(int));
|
|
|
|
nfr = factx(n, r+1);
|
|
nrf = factx(n-r, 1);
|
|
combi->total = (int)(nfr / nrf);
|
|
|
|
reset_combi(combi);
|
|
return combi;
|
|
}
|
|
|
|
/* returns NULL when we're done otherwise returns input. */
|
|
combi_ctx *next_combi(combi_ctx *combi)
|
|
{
|
|
int i = combi->r - 1, j;
|
|
|
|
if (combi->nleft == combi->total)
|
|
goto done;
|
|
else if (combi->nleft <= 0)
|
|
return NULL;
|
|
|
|
while (combi->a[i] == combi->n - combi->r + i)
|
|
i--;
|
|
combi->a[i] += 1;
|
|
for (j = i+1; j < combi->r; j++)
|
|
combi->a[j] = combi->a[i] + j - i;
|
|
|
|
done:
|
|
combi->nleft--;
|
|
return combi;
|
|
}
|
|
|
|
void free_combi(combi_ctx *combi)
|
|
{
|
|
sfree(combi->a);
|
|
sfree(combi);
|
|
}
|