mirror of
git://git.tartarus.org/simon/puzzles.git
synced 2025-04-20 23:51:29 -07:00
Refine drawing API semantics to pass drawing *
instead of void *
This changes the drawing API so that implementations receive a `drawing *` pointer with each call, instead of a `void *` pointer as they did previously. The `void *` context pointer has been moved to be a member of the `drawing` structure (which has been made public), from which it can be retrieved via the new `GET_HANDLE_AS_TYPE()` macro. To signal this breaking change to downstream front end authors, I've added a version number to the `drawing_api` struct, which will hopefully force them to notice. The motivation for this change is the upcoming introduction of a draw_polygon_fallback() function, which will use a series of calls to draw_line() to perform software polygon rasterization on platforms without a native polygon fill primitive. This function is fairly large, so I desired that it not be included in the binary distribution, except on platforms which require it (e.g. my Rockbox port). One way to achieve this is via link-time optimization (LTO, a.k.a. "interprocedural optimization"/IPO), so that the code is unconditionally compiled (preventing bit-rot) but only included in the linked executable if it is actually referenced from elsewhere. Practically, this precludes the otherwise straightforward route of including a run-time check of the `draw_polygon` pointer in the drawing.c middleware. Instead, Simon recommended that a front end be able to set its `draw_polygon` field to point to draw_polygon_fallback(). However, the old drawing API's semantics of passing a `void *` pointer prevented this from working in practice, since draw_polygon_fallback(), implemented in middleware, would not be able to perform any drawing operations without a `drawing *` pointer; with the new API, this restriction is removed, clearing the way for that function's introduction. This is a breaking change for front ends, which must update their implementations of the drawing API to conform. The migration process is fairly straightforward: every drawing API function which previously took a `void *` context pointer should be updated to take a `drawing *` pointer in its place. Then, where each such function would have previously casted the `void *` pointer to a meaningful type, they now instead retrieve the context pointer from the `handle` field of the `drawing` structure. To make this transition easier, the `GET_HANDLE_AS_TYPE()` macro is introduced to wrap the context pointer retrieval (see below for usage). As an example, an old drawing API function implementation would have looked like this: void frontend_draw_func(void *handle, ...) { frontend *fe = (frontend *)handle; /* do stuff with fe */ } After this change, that function would be rewritten as: void frontend_draw_func(drawing *dr, ...) { frontend *fe = GET_HANDLE_AS_TYPE(dr, frontend); /* do stuff with fe */ } I have already made these changes to all the in-tree front ends, but out-of-tree front ends will need to follow the procedure outlined above. Simon pointed out that changing the drawing API function pointer signatures to take `drawing *` instead of `void *` results only in a compiler warning, not an outright error. Thus, I've introduced a version field to the beginning of the `drawing_api` struct, which will cause a compilation error and hopefully force front ends to notice this. This field should be set to 1 for now. Going forward, it will provide a clear means of communicating future breaking API changes.
This commit is contained in:
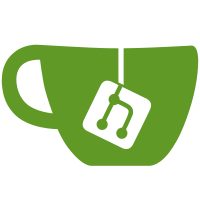
committed by
Simon Tatham
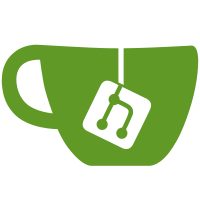
parent
a993fd45eb
commit
f37913002a
63
osx.m
63
osx.m
@ -1507,10 +1507,10 @@ struct frontend {
|
||||
/*
|
||||
* Drawing routines called by the midend.
|
||||
*/
|
||||
static void osx_draw_polygon(void *handle, const int *coords, int npoints,
|
||||
static void osx_draw_polygon(drawing *dr, const int *coords, int npoints,
|
||||
int fillcolour, int outlinecolour)
|
||||
{
|
||||
frontend *fe = (frontend *)handle;
|
||||
frontend *fe = GET_HANDLE_AS_TYPE(dr, frontend);
|
||||
NSBezierPath *path = [NSBezierPath bezierPath];
|
||||
int i;
|
||||
|
||||
@ -1536,10 +1536,10 @@ static void osx_draw_polygon(void *handle, const int *coords, int npoints,
|
||||
[fe->colours[outlinecolour] set];
|
||||
[path stroke];
|
||||
}
|
||||
static void osx_draw_circle(void *handle, int cx, int cy, int radius,
|
||||
static void osx_draw_circle(drawing *dr, int cx, int cy, int radius,
|
||||
int fillcolour, int outlinecolour)
|
||||
{
|
||||
frontend *fe = (frontend *)handle;
|
||||
frontend *fe = GET_HANDLE_AS_TYPE(dr, frontend);
|
||||
NSBezierPath *path = [NSBezierPath bezierPath];
|
||||
|
||||
[[NSGraphicsContext currentContext] setShouldAntialias:YES];
|
||||
@ -1559,9 +1559,9 @@ static void osx_draw_circle(void *handle, int cx, int cy, int radius,
|
||||
[fe->colours[outlinecolour] set];
|
||||
[path stroke];
|
||||
}
|
||||
static void osx_draw_line(void *handle, int x1, int y1, int x2, int y2, int colour)
|
||||
static void osx_draw_line(drawing *dr, int x1, int y1, int x2, int y2, int colour)
|
||||
{
|
||||
frontend *fe = (frontend *)handle;
|
||||
frontend *fe = GET_HANDLE_AS_TYPE(dr, frontend);
|
||||
NSBezierPath *path = [NSBezierPath bezierPath];
|
||||
NSPoint p1 = { x1 + 0.5, fe->h - y1 - 0.5 };
|
||||
NSPoint p2 = { x2 + 0.5, fe->h - y2 - 0.5 };
|
||||
@ -1579,12 +1579,12 @@ static void osx_draw_line(void *handle, int x1, int y1, int x2, int y2, int colo
|
||||
}
|
||||
|
||||
static void osx_draw_thick_line(
|
||||
void *handle, float thickness,
|
||||
drawing *dr, float thickness,
|
||||
float x1, float y1,
|
||||
float x2, float y2,
|
||||
int colour)
|
||||
{
|
||||
frontend *fe = (frontend *)handle;
|
||||
frontend *fe = GET_HANDLE_AS_TYPE(dr, frontend);
|
||||
NSBezierPath *path = [NSBezierPath bezierPath];
|
||||
|
||||
assert(colour >= 0 && colour < fe->ncolours);
|
||||
@ -1597,9 +1597,9 @@ static void osx_draw_thick_line(
|
||||
[path stroke];
|
||||
}
|
||||
|
||||
static void osx_draw_rect(void *handle, int x, int y, int w, int h, int colour)
|
||||
static void osx_draw_rect(drawing *dr, int x, int y, int w, int h, int colour)
|
||||
{
|
||||
frontend *fe = (frontend *)handle;
|
||||
frontend *fe = GET_HANDLE_AS_TYPE(dr, frontend);
|
||||
NSRect r = { {x, fe->h - y - h}, {w,h} };
|
||||
|
||||
[[NSGraphicsContext currentContext] setShouldAntialias:NO];
|
||||
@ -1609,11 +1609,11 @@ static void osx_draw_rect(void *handle, int x, int y, int w, int h, int colour)
|
||||
|
||||
NSRectFill(r);
|
||||
}
|
||||
static void osx_draw_text(void *handle, int x, int y, int fonttype,
|
||||
static void osx_draw_text(drawing *dr, int x, int y, int fonttype,
|
||||
int fontsize, int align, int colour,
|
||||
const char *text)
|
||||
{
|
||||
frontend *fe = (frontend *)handle;
|
||||
frontend *fe = GET_HANDLE_AS_TYPE(dr, frontend);
|
||||
NSString *string = [NSString stringWithUTF8String:text];
|
||||
NSDictionary *attr;
|
||||
NSFont *font;
|
||||
@ -1646,7 +1646,7 @@ static void osx_draw_text(void *handle, int x, int y, int fonttype,
|
||||
|
||||
[string drawAtPoint:point withAttributes:attr];
|
||||
}
|
||||
static char *osx_text_fallback(void *handle, const char *const *strings,
|
||||
static char *osx_text_fallback(drawing *dr, const char *const *strings,
|
||||
int nstrings)
|
||||
{
|
||||
/*
|
||||
@ -1660,7 +1660,7 @@ struct blitter {
|
||||
int x, y;
|
||||
NSImage *img;
|
||||
};
|
||||
static blitter *osx_blitter_new(void *handle, int w, int h)
|
||||
static blitter *osx_blitter_new(drawing *dr, int w, int h)
|
||||
{
|
||||
blitter *bl = snew(blitter);
|
||||
bl->x = bl->y = -1;
|
||||
@ -1669,14 +1669,14 @@ static blitter *osx_blitter_new(void *handle, int w, int h)
|
||||
bl->img = [[NSImage alloc] initWithSize:NSMakeSize(w, h)];
|
||||
return bl;
|
||||
}
|
||||
static void osx_blitter_free(void *handle, blitter *bl)
|
||||
static void osx_blitter_free(drawing *dr, blitter *bl)
|
||||
{
|
||||
[bl->img release];
|
||||
sfree(bl);
|
||||
}
|
||||
static void osx_blitter_save(void *handle, blitter *bl, int x, int y)
|
||||
static void osx_blitter_save(drawing *dr, blitter *bl, int x, int y)
|
||||
{
|
||||
frontend *fe = (frontend *)handle;
|
||||
frontend *fe = GET_HANDLE_AS_TYPE(dr, frontend);
|
||||
int sx, sy, sX, sY, dx, dy, dX, dY;
|
||||
[fe->image unlockFocus];
|
||||
[bl->img lockFocus];
|
||||
@ -1718,9 +1718,9 @@ static void osx_blitter_save(void *handle, blitter *bl, int x, int y)
|
||||
bl->x = x;
|
||||
bl->y = y;
|
||||
}
|
||||
static void osx_blitter_load(void *handle, blitter *bl, int x, int y)
|
||||
static void osx_blitter_load(drawing *dr, blitter *bl, int x, int y)
|
||||
{
|
||||
frontend *fe = (frontend *)handle;
|
||||
frontend *fe = GET_HANDLE_AS_TYPE(dr, frontend);
|
||||
if (x == BLITTER_FROMSAVED && y == BLITTER_FROMSAVED) {
|
||||
x = bl->x;
|
||||
y = bl->y;
|
||||
@ -1729,14 +1729,14 @@ static void osx_blitter_load(void *handle, blitter *bl, int x, int y)
|
||||
fromRect:NSMakeRect(0, 0, bl->w, bl->h)
|
||||
operation:NSCompositeCopy fraction:1.0];
|
||||
}
|
||||
static void osx_draw_update(void *handle, int x, int y, int w, int h)
|
||||
static void osx_draw_update(drawing *dr, int x, int y, int w, int h)
|
||||
{
|
||||
frontend *fe = (frontend *)handle;
|
||||
frontend *fe = GET_HANDLE_AS_TYPE(dr, frontend);
|
||||
[fe->view setNeedsDisplayInRect:NSMakeRect(x, fe->h - y - h, w, h)];
|
||||
}
|
||||
static void osx_clip(void *handle, int x, int y, int w, int h)
|
||||
static void osx_clip(drawing *dr, int x, int y, int w, int h)
|
||||
{
|
||||
frontend *fe = (frontend *)handle;
|
||||
frontend *fe = GET_HANDLE_AS_TYPE(dr, frontend);
|
||||
NSRect r = { {x, fe->h - y - h}, {w, h} };
|
||||
|
||||
if (!fe->clipped)
|
||||
@ -1744,31 +1744,32 @@ static void osx_clip(void *handle, int x, int y, int w, int h)
|
||||
[NSBezierPath clipRect:r];
|
||||
fe->clipped = true;
|
||||
}
|
||||
static void osx_unclip(void *handle)
|
||||
static void osx_unclip(drawing *dr)
|
||||
{
|
||||
frontend *fe = (frontend *)handle;
|
||||
frontend *fe = GET_HANDLE_AS_TYPE(dr, frontend);
|
||||
if (fe->clipped)
|
||||
[[NSGraphicsContext currentContext] restoreGraphicsState];
|
||||
fe->clipped = false;
|
||||
}
|
||||
static void osx_start_draw(void *handle)
|
||||
static void osx_start_draw(drawing *dr)
|
||||
{
|
||||
frontend *fe = (frontend *)handle;
|
||||
frontend *fe = GET_HANDLE_AS_TYPE(dr, frontend);
|
||||
[fe->image lockFocus];
|
||||
fe->clipped = false;
|
||||
}
|
||||
static void osx_end_draw(void *handle)
|
||||
static void osx_end_draw(drawing *dr)
|
||||
{
|
||||
frontend *fe = (frontend *)handle;
|
||||
frontend *fe = GET_HANDLE_AS_TYPE(dr, frontend);
|
||||
[fe->image unlockFocus];
|
||||
}
|
||||
static void osx_status_bar(void *handle, const char *text)
|
||||
static void osx_status_bar(drawing *dr, const char *text)
|
||||
{
|
||||
frontend *fe = (frontend *)handle;
|
||||
frontend *fe = GET_HANDLE_AS_TYPE(dr, frontend);
|
||||
[fe->window setStatusLine:text];
|
||||
}
|
||||
|
||||
const struct drawing_api osx_drawing = {
|
||||
1,
|
||||
osx_draw_text,
|
||||
osx_draw_rect,
|
||||
osx_draw_line,
|
||||
|
Reference in New Issue
Block a user