mirror of
git://git.tartarus.org/simon/puzzles.git
synced 2025-04-20 23:51:29 -07:00
Files
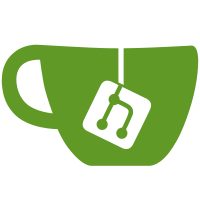
The big mathematical news this month is that a polygon has been discovered that will tile the plane but only aperiodically. Penrose tiles achieve this with two tile types; it's been an open question for decades whether you could do it with only one tile. Now someone has announced the discovery of such a thing, so _obviously_ this mathematically exciting tiling ought to be one of the Loopy grid options! The polygon, named a 'hat' by its discoverers, consists of the union of eight cells of the 'Kites' periodic tiling that Loopy already implements. So all the vertex coordinates of the whole tiling are vertices of the Kites grid, which makes handling the coordinates in an exact manner a lot easier than Penrose tilings. What's _harder_ than Penrose tilings is that, although this tiling can be generated by a vaguely similar system of recursive expansion, the expansion is geometrically distorting, which means you can't easily figure out which tiles can be discarded early to save CPU. Instead I've come up with a completely different system for generating a patch of tiling, by using a hierarchical coordinate system to track a location within many levels of the expansion process without ever simulating the process as a whole. I'm really quite pleased with that technique, and am tempted to try switching the Penrose generator over to it too - except that we'd have to keep the old generator around to stop old game ids being invalidated, and also, I think it would be slightly trickier without an underlying fixed grid and without overlaps in the tile expansion system. However, before coming up with that, I got most of the way through implementing the more obvious system of actually doing the expansions. The result worked, but was very slow (because I changed approach rather than try to implement tree-pruning under distortion). But the code was reusable for two other useful purposes: it generated the lookup tables needed for the production code, and it also generated a lot of useful diagrams. So I've committed it anyway as a supporting program, in a new 'aux' source subdirectory, and in aux/doc is a writeup of the coordinate system's concepts, with all those diagrams. (That's the kind of thing I'd normally put in a huge comment at the top of the file, but doing all those diagrams in ASCII art would be beyond miserable.) From a gameplay perspective: the hat polygon has 13 edges, but one of them has a vertex of the Kites tiling in the middle, and sometimes two other tile boundaries meet at that vertex. I've chosen to represent every hat as having degree 14 for Loopy purposes, because if you only included that extra vertex when it was needed, then people would be forever having to check whether this was a 13-hat or a 14-hat and it would be nightmarish to play. Even so, there's a lot of clicking involved to turn all those fiddly individual edges on or off. This grid is noticeably nicer to play in 'autofollow' mode, by setting LOOPY_AUTOFOLLOW in the environment to either 'fixed' or 'adaptive'. I'm tempted to make 'fixed' the default, except that I think it would confuse players of ordinary square Loopy!
68 lines
2.7 KiB
C
68 lines
2.7 KiB
C
#ifndef PUZZLES_HAT_H
|
|
#define PUZZLES_HAT_H
|
|
|
|
struct HatPatchParams {
|
|
/*
|
|
* A patch of hat tiling is identified by giving the coordinates
|
|
* of the kite in one corner, using a multi-level coordinate
|
|
* system based on metatile expansions. Coordinates are a sequence
|
|
* of small non-negative integers. The valid range for each
|
|
* coordinate depends on the next coordinate, or on final_metatile
|
|
* if it's the last one in the list. The largest valid range is
|
|
* {0,...,12}.
|
|
*
|
|
* 'final_metatile' is one of the characters 'H', 'T', 'P' or 'F'.
|
|
*/
|
|
size_t ncoords;
|
|
unsigned char *coords;
|
|
char final_metatile;
|
|
};
|
|
|
|
/*
|
|
* Fill in HatPatchParams with a randomly selected set of coordinates,
|
|
* in enough detail to generate a patch of tiling covering an area of
|
|
* w x h 'squares' of a kite tiling.
|
|
*
|
|
* The kites grid is considered to be oriented so that it includes
|
|
* horizontal lines and not vertical ones. So each of the smallest
|
|
* equilateral triangles in the grid has a bounding rectangle whose
|
|
* height is sqrt(3)/2 times its width, and either the top or the
|
|
* bottom of that bounding rectangle is the horizontal edge of the
|
|
* triangle. A 'square' of a kite tiling (for convenience of choosing
|
|
* grid dimensions) counts as one of those bounding rectangles.
|
|
*
|
|
* The 'coords' field of the structure will be filled in with a new
|
|
* dynamically allocated array. Any previous pointer in that field
|
|
* will be overwritten.
|
|
*/
|
|
void hat_tiling_randomise(struct HatPatchParams *params, int w, int h,
|
|
random_state *rs);
|
|
|
|
/*
|
|
* Validate a HatPatchParams to ensure it contains no illegal
|
|
* coordinates. Returns NULL if it's acceptable, or an error string if
|
|
* not.
|
|
*/
|
|
const char *hat_tiling_params_invalid(const struct HatPatchParams *params);
|
|
|
|
/*
|
|
* Generate the actual set of hat tiles from a HatPatchParams, passing
|
|
* each one to a callback. The callback receives the vertices of each
|
|
* point, as a sequence of 2*nvertices integers, with x,y coordinates
|
|
* interleaved.
|
|
*
|
|
* The x coordinates are measured in units of 1/4 of the side length
|
|
* of the smallest equilateral triangle, or equivalently, 1/2 the
|
|
* length of one of the long edges of a single kite. The y coordinates
|
|
* are measured in units of 1/6 the height of the triangle, which is
|
|
* also 1/2 the length of the short edge of a kite. Therefore, you can
|
|
* expect x to go up to 4*w and y up to 6*h.
|
|
*/
|
|
typedef void (*hat_tile_callback_fn)(void *ctx, size_t nvertices,
|
|
int *coords);
|
|
|
|
void hat_tiling_generate(const struct HatPatchParams *params, int w, int h,
|
|
hat_tile_callback_fn cb, void *cbctx);
|
|
|
|
#endif
|